From Spaghetti to Sushi: Writing Clean and Maintainable React Code
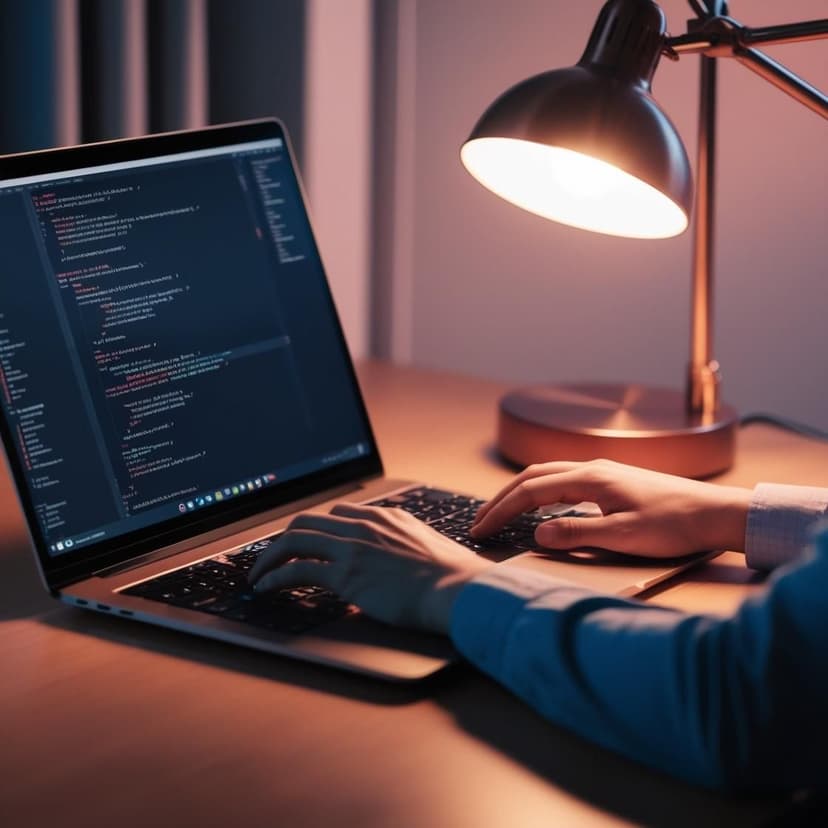
Jonathan Bracho
Aug 05, 2024
Picture this: you're handed a React codebase. The folder structure is a maze, the components resemble a teenager's bedroom, and prop drilling goes deeper than the Mariana Trench. We've all been there. If messy code is the spaghetti of our digital kitchen, clean, maintainable code is the sushi—artful, precise, and satisfying.
Over my 4.5+ years as a Frontend Developer, I’ve had my fair share of chaotic kitchens (read: codebases). Transforming them into elegant, maintainable sushi platters has been both a challenge and a joy. Let me share some tips, tricks, and a few bruises from my journey to help you elevate your React code from "boiled noodles" to Michelin-star-worthy.
The Mise en Place: Setting Up for Success.
In a sushi chef's world, mise en place means "everything in its place." For developers, this starts with project setup.
- Modular File Structures.
Group related files together. A components folder bursting with hundreds of files? That’s a code smell. Instead, structure your project around features or domains (features/Blog, features/Auth). Bonus: future you will thank you when debugging.
- TypeScript Is Your Soy Sauce.
You can live without it, but why would you? TypeScript catches bugs early and makes refactoring less scary. Invest time in defining interfaces and types, even for your props. Your teammates (and IDE) will adore you for it.
Rolling the Perfect Maki: Component Best Practices.
A messy roll falls apart. The same goes for components.
- Keep Components Small.
If your component exceeds 300 lines, it’s time for some sashimi (slice it up). Smaller, single-responsibility components are easier to read, test, and reuse.
- Prop Drilling vs. Context vs. Zustand.
Prop drilling is like passing ingredients hand-to-hand across the kitchen—messy and slow. For state that’s deeply nested but specific to a section, use React's Context API or libraries like Zustand. For global state, Redux Toolkit or Zustand's simple API can save your sanity.
- Avoid Side Dish Chaos.
Handle side effects cleanly. React Query is your best friend for data fetching, while hooks like useEffect should remain focused and concise. Never overburden lifecycle methods—they’re not your catch-all drawer.
Precision Cuts: Clean Code Practices.
Good sushi demands precision. So does maintainable code.
- Clear Naming Conventions.
Avoid generic names like handleClick. Be specific: handleAddToCart. Code should be self-documenting—comments shouldn’t have to explain what a variable named -x- does.
- Reusable Hooks.
If you’ve written the same logic in two places, congratulations—you’ve got a candidate for a custom hook. Hooks like usePagination or useAuth encapsulate logic and keep your components lean.
- Styled-Components vs. TailwindCSS vs. SCSS.
Pick a styling approach and stick to it. Mixing methodologies leads to chaos. Personally, I lean on SCSS for complex designs and TailwindCSS for rapid prototyping. But whatever you choose, keep your styles scoped and avoid the dreaded !important.
Plate Presentation: Testing and Documentation.
Even the best sushi can fail if it’s served poorly.
- Jest and React Testing Library.
Unit tests and integration tests aren’t optional—they’re your wasabi and pickled ginger. They might sting to write at first, but they cleanse the palate when debugging.
- Storybook
Document your components visually. Not only does this help your team, but it also gives you a sense of pride seeing your component library grow into a beautiful menu.
- CI/CD Integration
Automate testing and linting. Git hooks with tools like Husky can ensure no raw fish (i.e., untested code) makes it to production.
Personal Anecdote: The Sushi Crisis.
I once inherited a codebase that resembled the contents of a junk drawer—hardcoded API URLs, global state for everything (even the hamburger menu), and stylesheets named styles1.css, styles2.css. It was chaos.
The turning point came when I introduced modularization. We reorganized the file structure, converted constants to enums, and rewrote styles in SCSS with proper class names. The result? A codebase as clean as a sushi bar—and a team that stopped dreading PR reviews.
Final Thoughts: The Zen of Code.
Clean code is like sushi—it looks simple, but achieving that simplicity takes skill, patience, and discipline. By focusing on maintainability, testing, and organization, you can turn any spaghetti codebase into a sushi masterpiece.
So, next time you face a messy React project, channel your inner sushi chef. Take a deep breath, sharpen your tools, and roll with precision. The result will be as satisfying as biting into perfectly rolled sushi—your teammates will thank you, and your future self will toast you with sake.
Happy coding, or as the sushi chefs say, Itadakimasu!